Handling Code Conflicts on the Same Branch in Git
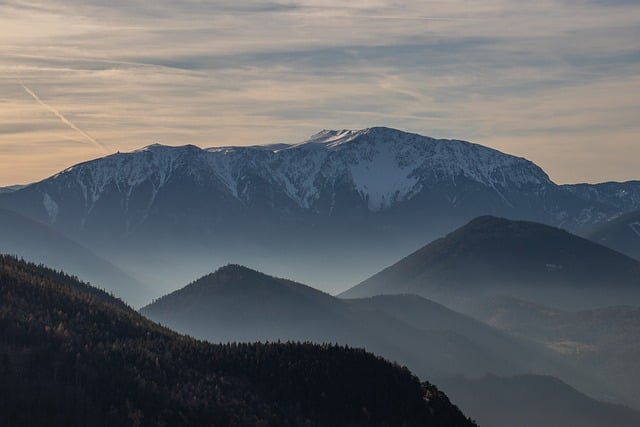
Scenario Description
Let’s assume we have a Git repository with a main
branch and a file called example.txt
with the following content:
1 | Hello, this is a demo file. |
Both Developer A and Developer B are working on the main
branch and make changes to the same part of example.txt
at the same time.
Step 1: Actions by Developer A
Developer A modifies the example.txt
file by adding a new line:
1 | Hello, this is a demo file. |
Developer A then commits the change and pushes it to the remote repository:
1 | git add example.txt |
At this point, Developer A’s changes have been successfully pushed to the remote repository.
Step 2: Actions by Developer B
Meanwhile, Developer B also makes a local modification to the same example.txt
file, adding a different change:
1 | Hello, this is a demo file. |
Developer B commits the change:
1 | git add example.txt |
Next, Developer B tries to push the changes to the remote repository:
1 | git push origin main |
Step 3: Conflict Occurs
At this point, Git detects that there are new changes in the remote main
branch (the changes Developer A has already pushed) and that Developer B’s changes conflict with those changes. Git will inform Developer B that they need to pull the latest changes from the remote repository before pushing:
1 | To [remote-repo] |
Developer B now needs to execute a git pull
to fetch the latest changes from the remote repository:
1 | git pull origin main |
When Git attempts to merge the changes, it will detect a conflict in example.txt
because both Developer A and Developer B modified the same part of the file. The following message will appear, indicating the conflict:
1 | Auto-merging example.txt |
At this point, if Developer B opens the example.txt
file, they will see the conflict markers indicating the different changes:
1 | Hello, this is a demo file. |
Here, <<<<<<< HEAD
represents Developer B’s local changes, while =======
and >>>>>>> [commit-id]
represent the changes from the remote repository (Developer A’s changes).
Resolving the Conflict
Option 1: Manually Resolving the Conflict
Developer B needs to manually edit the example.txt
file to resolve the conflict. For example, they could merge both changes by modifying the file as follows:
1 | Hello, this is a demo file. |
After saving the file, Developer B should then mark the conflict as resolved and commit the changes:
1 | git add example.txt |
Finally, Developer B can successfully push the changes to the remote repository:
1 | git push origin main |
Option 2: Discarding Local Changes
If Developer B decides to discard their local changes and use the remote version of the file (the changes from Developer A), they can use the following command:
1 | git reset --hard origin/main |
This command will discard Developer B’s unpushed changes and reset the local code to match the remote repository’s state. Afterward, Developer B can continue working or make new changes.
Summary
A conflict on the same branch (such as main
) often occurs when multiple developers are working on the same file and edit the same section of code. There are typically two ways to resolve these conflicts: 1. Manually resolve the conflict: Edit the conflicted file to merge the changes. 2. Discard local changes: If the local changes are not needed, discard them and use the remote version.