Java File 类
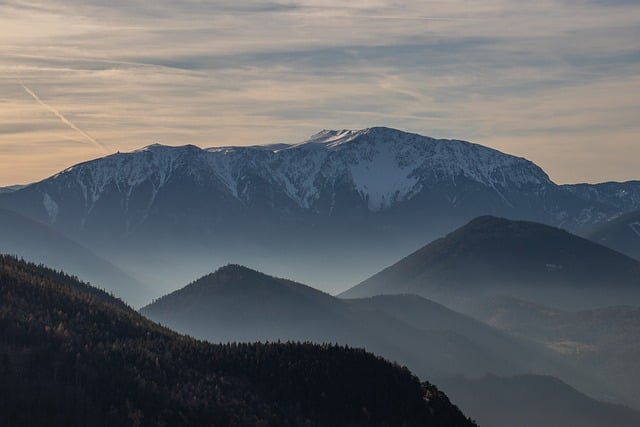
声明:这是一篇学习笔记,可能会有错误。如有错误,还请各位大神指出。
File 类是文件和目录路径名的抽象表示。
1 | public class File |
一般来说,我们想创建一个File类的实例,我们可以通过以下代码:
1 | File file = new File("a.txt"); |
但注意的是,这样并不会创建名为 a.txt 的文件。如果我们想创建文件我们需要使用下面的代码:
1 | File file = new File("a.txt"); |
以上代码,我们创建了一个File类的实例,名为file。之后我们调用File类的方法 createNewFile(),这个方法可能会抛出异常,所以我们需要用 try-catch 语句。同时这个方法会返回一个布尔值,如果指定的文件不存在且已成功创建,则返回true;如果指定的文件已存在则返回false。
注意的是,下面两行代码含义是一样的。
1 | File file = new File("a.txt"); |
创建出来的文件会出现在与工程项目中src文件夹同级的文件夹中。
构造方法
构造方法 | 描述 |
---|---|
File(File parent, String child) | 创建一个新的File类的实例,parent是File类型的父路径,child是子路径 |
File(String pathname) | 通过将一个字符串描述的路径,创建一个新的File类的实例 |
File(String parent, String child) | 创建一个新的File类的实例,parent是父路径,child是子路径 |
File(URI uri) | 通过将URI转换成抽象路径,创建一个新的File类的实例 |
前文中已经给出一个代码示例了,下面给出其他的几个。
File(File parent, String child)
1 | File parentPath = new File("./data"); |
File(String parent, String child)
1 | String parentPath = "./data"; |
File(URI uri)
1 | URI filePath = null; |
为了让以上代码创建成功,在项目文件夹我创建了一个文件夹名为 data, 其中放了一个文件 Art.txt
方法总结
前面已经描述了createNewFile()的使用,但是需要注意的一点是:
1 | File file = new File("./data/a.txt"); |
如果data文件夹不存在,会抛出IOException异常。
本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明来自 Vincent's Blog!